Modal (Bottom)
A component that displays content in a popup dialog anchored to the bottom of the screen.
Preview
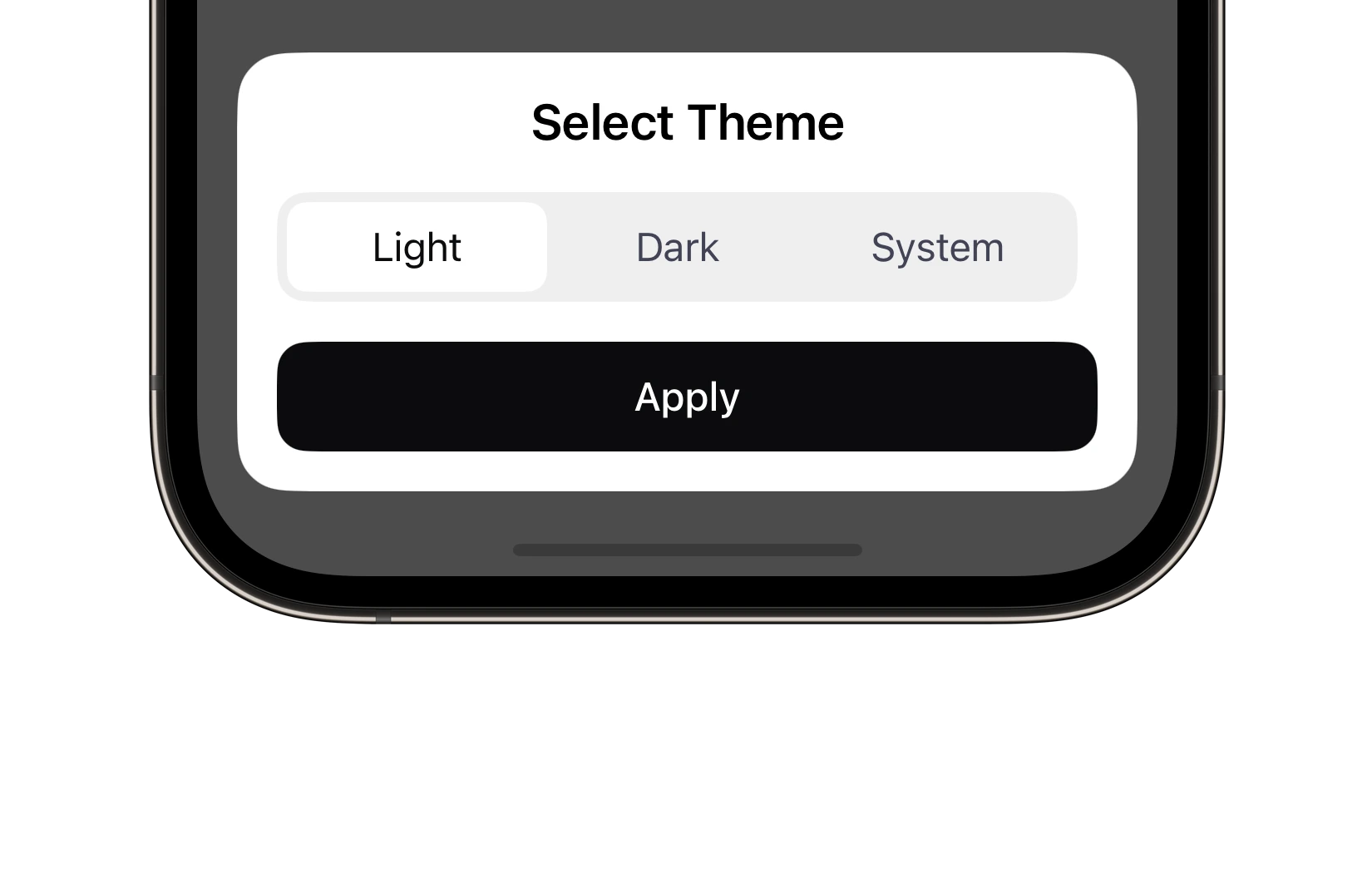
Code Example
API
BottomModalVM Props
A model that defines the appearance properties for a bottom modal component.
Name | Type | Default | Description |
---|---|---|---|
backgroundColor | UniversalColor? | nil | The background color of the modal. |
borderWidth | BorderWidth | small | The border thickness of the modal. |
closesOnOverlayTap | Bool | true | Indicates whether the modal should close when tapping on the overlay. |
contentPaddings | Paddings | padding: 16 | The padding applied to the modal's content area. |
contentSpacing | CGFloat | 16 | The spacing between header, body, and footer. |
cornerRadius | ContainerRadius | medium | The corner radius of the modal. |
hidesOnSwipe | Bool | true | Indicates whether the modal should hide when swiped down. |
isDraggable | Bool | true | Indicates whether the modal can be dragged vertically. |
overlayStyle | ModalOverlayStyle | dimmed | The style of the overlay displayed behind the modal. |
outerPaddings | Paddings | padding: 20 | The padding outside the modal content, creating space from screen edges. |
size | ModalSize | medium | The predefined maximum size of the modal. |
transition | ModalTransition | fast | The transition duration of modal's animations. |
SUBottomModal
- A SwiftUI view modifier that presents a bottom-aligned modal.
public func bottomModal<Header: View, Body: View, Footer: View>(
isPresented: Binding<Bool>,
model: BottomModalVM = .init(),
onDismiss: (() -> Void)? = nil,
@ViewBuilder header: @escaping () -> Header = { EmptyView() },
@ViewBuilder body: @escaping () -> Body,
@ViewBuilder footer: @escaping () -> Footer = { EmptyView() }
) -> some View
- A SwiftUI view modifier that presents a bottom-aligned modal bound to an optional identifiable item.
public func bottomModal<Item: Identifiable, Header: View, Body: View, Footer: View>(
item: Binding<Item?>,
model: @escaping (Item) -> BottomModalVM = { _ in .init() },
onDismiss: (() -> Void)? = nil,
@ViewBuilder header: @escaping (Item) -> Header,
@ViewBuilder body: @escaping (Item) -> Body,
@ViewBuilder footer: @escaping (Item) -> Footer
) -> some View
- A SwiftUI view modifier that presents a bottom-aligned modal bound to an optional identifiable item.
public func bottomModal<Item: Identifiable, Body: View>(
item: Binding<Item?>,
model: @escaping (Item) -> BottomModalVM = { _ in .init() },
onDismiss: (() -> Void)? = nil,
@ViewBuilder body: @escaping (Item) -> Body
) -> some View
UKBottomModalController
Initializers
public init(
model: BottomModalVM = .init(),
header: Content? = nil,
body: Content,
footer: Content? = nil
)
Public Properties
Name | Type | Description |
---|---|---|
model | BottomVM | A model that defines the appearance properties. |
Public Subviews
Name | Type | Description |
---|---|---|
header | UIView? | The optional header view of the modal. |
body | View | The main body view of the modal. |
footer | UIView? | The optional footer view of the modal. |
contentView | UIView | The content view, holding the header, body, and footer. |
bodyWrapper | UIScrollView | A scrollable wrapper for the body content. |
overlay | UIView | The overlay view that appears behind the modal. |