Segmented Control
A component that allows users to choose between multiple segments or options.
Preview
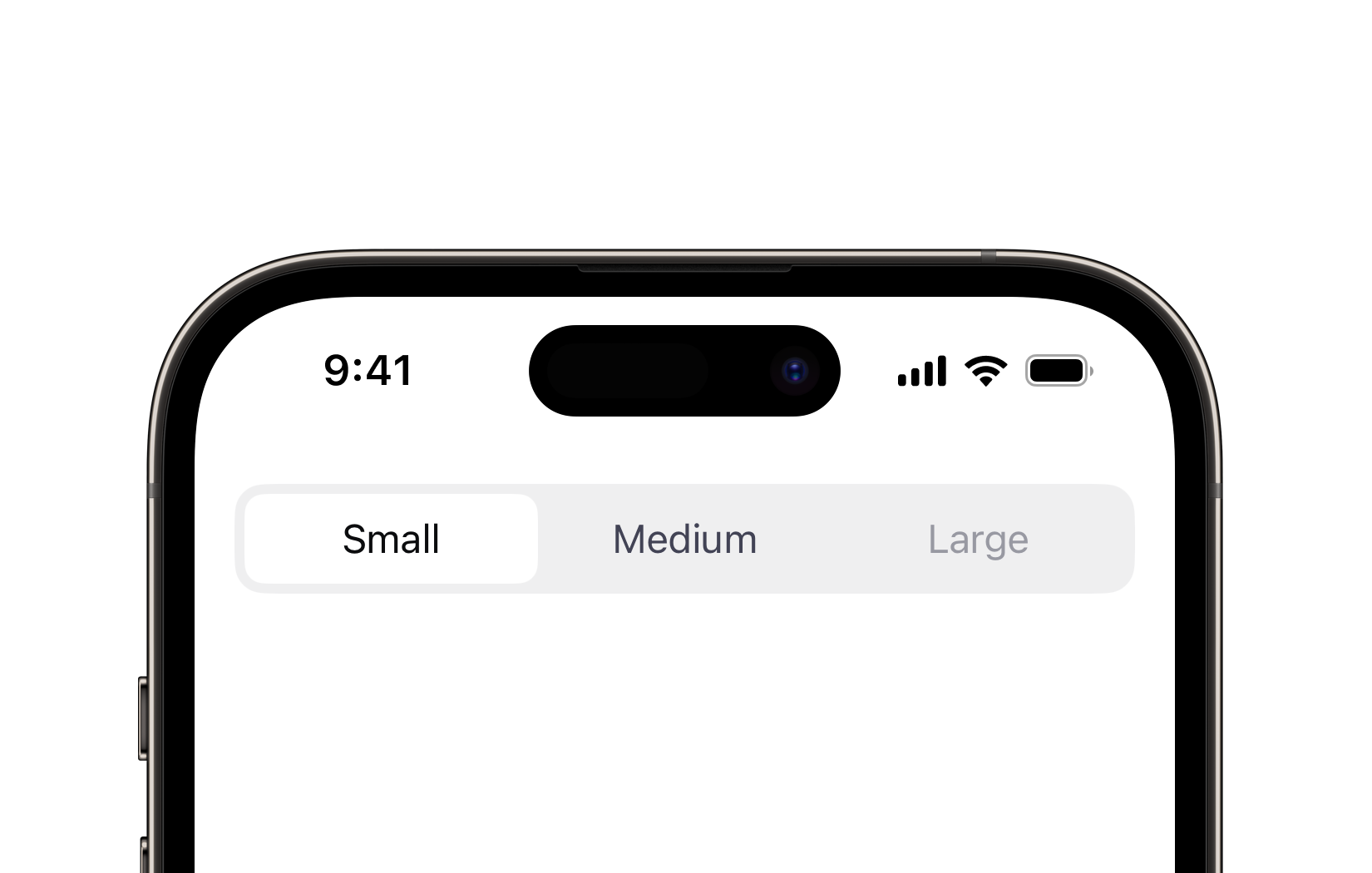
Code Example
API
SegmentedControlVM Props
A model that defines the appearance properties for a segmented control component.
Name | Type | Default | Description |
---|---|---|---|
color | ComponentColor? | nil | The color of the segmented control. |
cornerRadius | ComponentRadius | medium | The corner radius of the segmented control. |
font | UniversalFont? | nil | The font used for the segmented control items' titles. |
isEnabled | Bool | true | A Boolean value indicating whether the segmented control is enabled. |
isFullWidth | Bool | false | A Boolean value indicating whether the segmented control should take full width of its parent view. |
items | [SegmentedControlItemVM<ID>] | [] | The array of items in the segmented control. Must contain at least one item with unique identifiers. |
size | ComponentSize | medium | The predefined size of the segmented control. |
SegmentedControlItemVM Props
A model that defines the data and appearance properties for an item in a segmented control.
Name | Type | Default | Description |
---|---|---|---|
id | ID | — | The unique identifier for the segmented control item. |
title | String | "" | The text displayed for the segmented control item. |
font | UniversalFont? | nil | The font used for the item's title. |
isEnabled | Bool | true | A Boolean value indicating whether the item is enabled or disabled. |
SUSegmentedControl
public init(
selectedId: Binding<ID?>,
model: SegmentedControlVM<ID>
)
UKSegmentedControl
Initializers
public init(
selectedId: ID,
model: SegmentedControlVM<ID>,
onSelectionChange: @escaping (ID) -> Void = { _ in }
)
Public Properties
Name | Type | Description |
---|---|---|
onSelectionChange | (ID) -> Void | A closure that is triggered when a selected segment changes. |
model | SegmentedControlVM | A model that defines the appearance properties. |
selectedId | ID? | An identifier of the selected segment. |
Public Subviews
Name | Type | Description |
---|---|---|
container | UIView | A view that contains all segments. |
segments | [UKSegmentedControl.Segment] | An array of views that represent segments. |
selectedSegment | UIView | A view that highlights a selected segment. |